Creating Your First Application
In this section, we are not going into the technical aspects of VB programming; just have a feel of
it. Now, you can try out the examples below:
Example 2.1.1 is a simple program. First
of all, you have to launch Microsoft Visual Basic. Normally, a default form Form1 will be available for you to start
your new project. Now, double click on form1, the source code window for form1 as shown in figure 2.1 will appear. The top
of the source code window consists of a list of objects and their associated events or procedures. In figure 2.1, the object
displayed is Form and the associated procedure is Load.
When you click on the object
box, the drop-down list will display a list of objects you have inserted into your form as shown in figure 2.2. Here, you
can see a form, command button with the name Command1, a Label with the name Label1 and a PictureBox with the name Picture1.
Similarly, when you click on the procedure box, a list of procedures associated with the object will be displayed as shown
in figure 2.3. Some of the procedures associated with the object Form are Activate, Click, DblClick (which means Double-Click)
, DragDrop, keyPress and etc. Each object has its own set of procedures. You can always select an object and write codes for
any of its procedure in order to perform certain tasks.
You do not have to worry
about the beginning and the end statements (i.e. Private Sub Form_Load.......End Sub.); Just key in the lines in between the
above two statements exactly as are shown here. When you run the program, you will be surprise that nothing shown up .In order
to display the output of the program, you have to add the Form1.show statement like in Example 2.1.1 or you can just
use Form_Activate ( ) event procedure as shown in example 2.1.2. The command Print does not mean printing using a printer
but it means displaying the output on the computer screen. Now, press F5 or click on the run button to run the program and
you will get the output as shown in figure 2.4.
You can also
perform simple arithmetic calculations as shown in example 2.1.2. VB uses * to denote the multiplication operator and
/ to denote the division operator. The output is shown in figure 2.3, where the results are arranged vertically.
Figure 2.1 Source Code Window
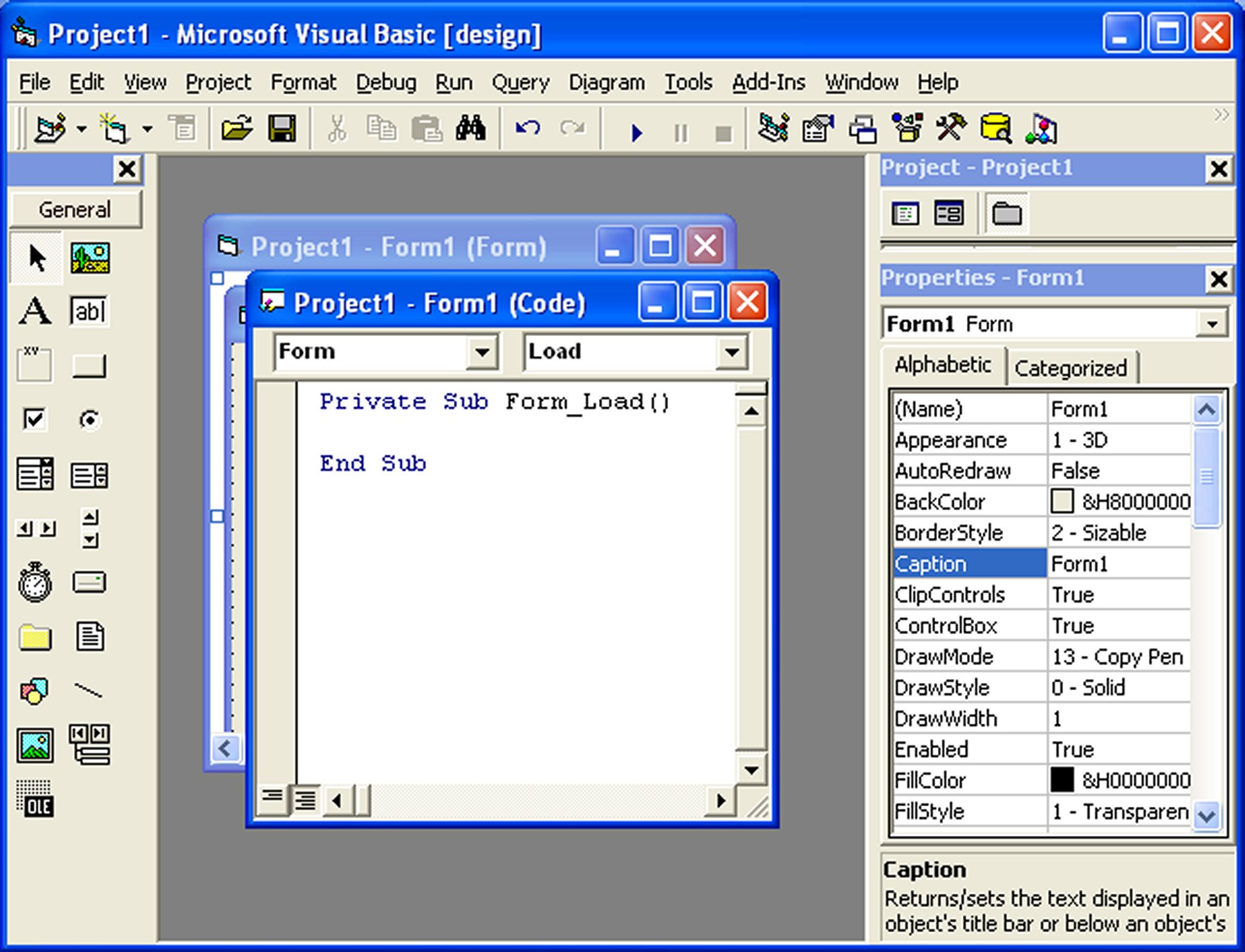
Figure 2.2: List of Objects
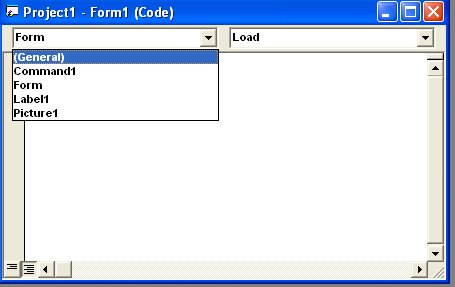 |
Figure 2.2: List of Procedures
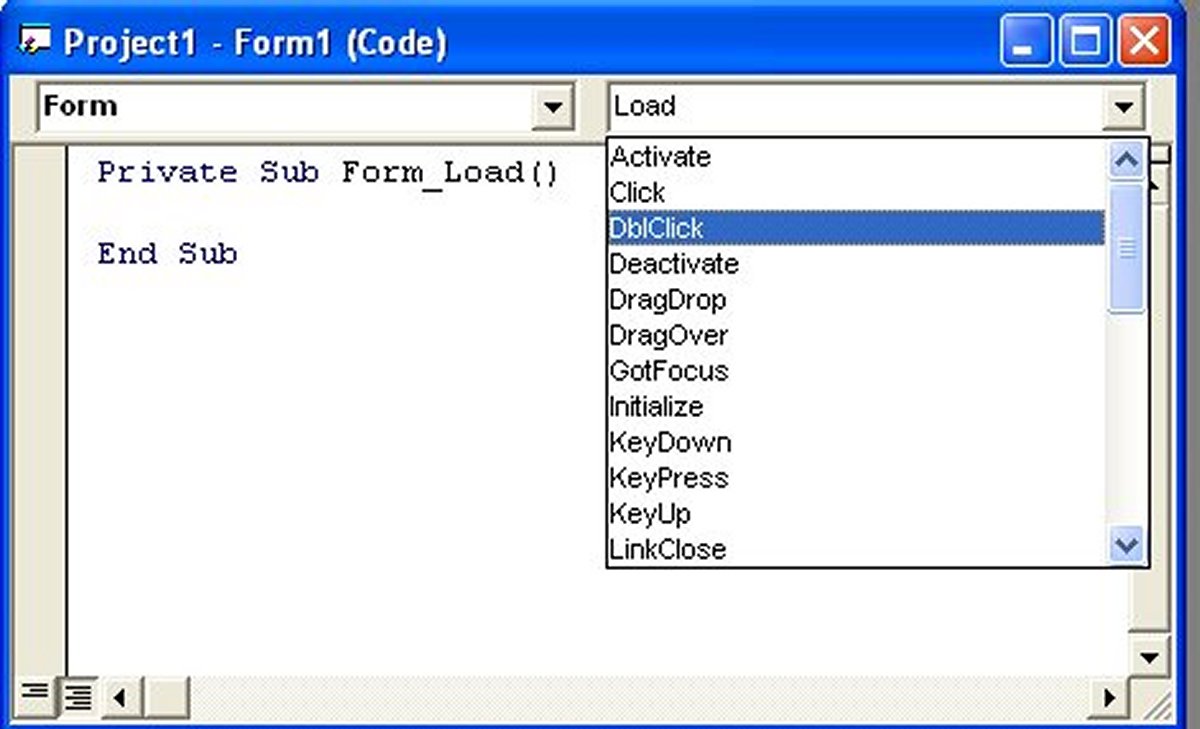
|
Example 2.1.1
Private
Sub Form_Load ( )
Form1.show
Print
“Welcome to Visual Basic tutorial”
End
Sub
|
Figure 2.4 : The output of example 2.1.1
|
Example 2.1.2
Private
Sub Form_Activate ( )
Print
20 + 10 Print 20 - 10 Print 20 * 10 Print 20 / 10
End
Sub
|
Figure 2.5: The output of example 2.1.2
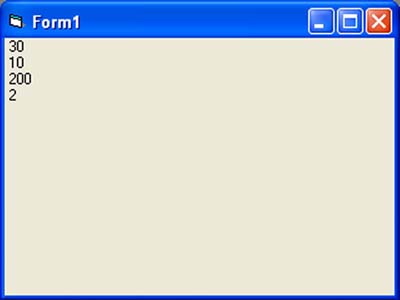
|
You can also use the + or
the & operator to join two or more texts (string) together like in example 2.1.4 (a) and (b)
Example 2.1.4(a)
Private
Sub A = Tom B = “likes" C = “to" D = “eat" E = “burger" Print A + B + C +
D + E |
Example 2.1.4(b)
Private
Sub A = Tom B = “likes" C = “to" D = “eat" E = “burger" Print A & B &
C & D & E |
The Output of Example 2.1.4(a)
&(b) is as shown in Figure 2.7.
2.2
Steps in Building a Visual Basic Application
Generally, there are three
basic steps in building a VB application. The steps are as follows:
Step 1 : Design the
interface
Step 2 : Set Properties of the controls (Objects)
Step 3 : Write the events' procedures