To be able to access and manage a database, you need to connect the ADO data control
to a database file. We are going to use BIBLIO.MDB that comes with VB6. To connect ADO to this database file , follow the
steps below:
a) Click on the ADO control on the form and open up the properties window.
b) Click on the ConnectionString property, the following dialog box will appear.
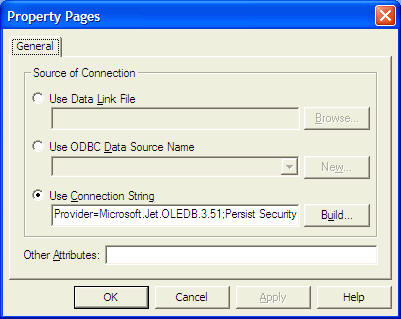
when the dialog box appear, select the Use Connection String's Option. Next, click
build and at the Data Link dialog box, double-Click the option labeled Microsoft Jet 3.51 OLE DB provider.
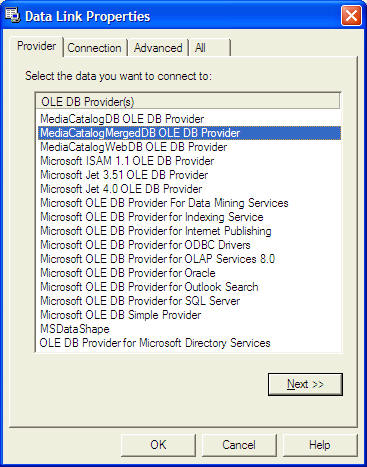
After that, click the Next button to select the file BIBLO.MDB. You can click
on Text Connection to ensure proper connection of the database file. Click OK to finish the connection.
Finally, click on the RecordSource property and set the command type to adCmd Table
and Table name to Titles. Now you are really to use the database file.
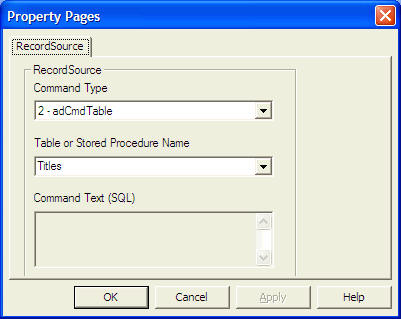
กก
Now, you need to write code for all the command buttons. After which, you can make
the ADO control invisible.
กก
กก
For the Save button, the program codes are as follow:
Private Sub cmdSave_Click()
adoBooks.Recordset.Fields("Title") = txtTitle.Text
adoBooks.Recordset.Fields("Year
Published") = txtPub.Text
adoBooks.Recordset.Fields("ISBN") = txtISBN.Text
adoBooks.Recordset.Fields("PubID") = txtPubID.Text
adoBooks.Recordset.Fields("Subject")
= txtSubject.Text
adoBooks.Recordset.Update
End Sub
For the Add button, the program codes are as follow:
Private Sub cmdAdd_Click()
adoBooks.Recordset.AddNew
End Sub
For the Delete button, the program codes are as follow:
Private Sub cmdDelete_Click()
Confirm = MsgBox("Are you sure you want to delete
this record?", vbYesNo, "Deletion Confirmation")
If Confirm = vbYes Then
adoBooks.Recordset.Delete
MsgBox "Record
Deleted!", , "Message"
Else
MsgBox "Record Not Deleted!", , "Message"
End If
End Sub
กก
For the Cancel button, the program codes are as follow:
Private Sub cmdCancel_Click()
txtTitle.Text = ""
txtPub.Text = ""
txtPubID.Text
= ""
txtISBN.Text = ""
txtSubject.Text = ""
End Sub
For the Previous (<) button, the program codes are
Private Sub cmdPrev_Click()
If Not adoBooks.Recordset.BOF Then
adoBooks.Recordset.MovePrevious
If
adoBooks.Recordset.BOF Then
adoBooks.Recordset.MoveNext
End If
End If
End Sub
For the Next(>) button,
the program codes are
Private Sub cmdNext_Click()
If Not adoBooks.Recordset.EOF Then
adoBooks.Recordset.MoveNext
If
adoBooks.Recordset.EOF Then
adoBooks.Recordset.MovePrevious
End If
End If
End Sub
TextBox2 DataField |
Year Published |
TextBox2 DataSource |
adoBooks |
TextBox3 Name |
txtISBN |
TextBox3 DataField |
ISBN |
TextBox3 DataSource |
adoBooks |
TextBox4 Name |
txtPubID |
TextBox4 DataField |
PubID |
TextBox4 DataSource |
adoBooks |
TextBox5 Name |
txtSubject |
TextBox5 DataField |
Subject |
TextBox5 DataSource |
adoBooks |
Command Button1 Name |
cmdSave |
Command Button1 Caption |
&Save |
Command Button2 Name |
cmdAdd |
Command Button2 Caption |
&Add |
Command Button3 Name |
cmdDelete |
Command Button3 Caption |
&Delete |
Command Button4 Name |
cmdCancel |
Command Button4 Caption |
&Cancel |
Command Button5 Name |
cmdPrev |
Command Button5 Caption |
&< |
Command Button6 Name |
cmdNext |
Command Button6 Caption |
&> |
Command Button7 Name |
cmdExit |
Command Button7 Caption |
E&xit |